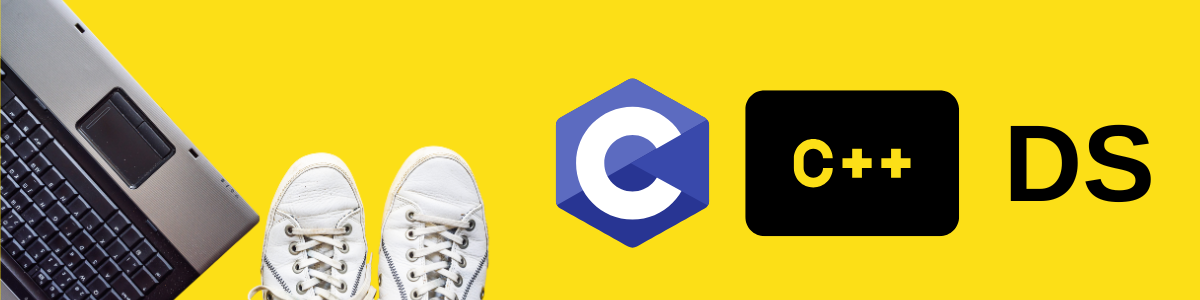
C Programming
C Programming is one of the foundations in Information Technology and Computer Science and most widely used Computer Language. It is very important for a Student and for working professional to know about C programming.
If anyone wants to start their career in IT, it should be from C programming which helps to build Logic in programming. That's the reason in almost all the courses at MyTechFocus we start with the revision of C programming.
- What is C?
- Keywords
- Constants
- Data Types
- Header Files
- Understanding & Starting with Programming
- Operatorss
- If-Else
- Loops
- Switch-Case
- Functions
- Pointer (Will be covered in depth)
- Arrays
- String
- Structures
- File IO
- Recursion
- Dynamic Memory Allocation (Malloc, Calloc, Realloc, Free)
C++ Programming
C++ is an Object oriented language and widely used for competitive programming.
C++ course at MY TECH FOCUS covers OPP's concepts in detail with topics like function overloading, Inheritance, Polymorphism, Exceptional handling etc.
Understanding the OPP's concepts in C++ is very important to learn JAVA. That's the reason we first take revision of C++ before starting with JAVA course.
- What is C++?
- What is OOPS?
- Objects
- Class
- Understanding Programs
- Constructors and Destructors
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
- Overloading
- Overriding
- Virtual table and Virtual pointers
- Abstract class
- Friend Function
- Operator Overloading
- Shallow and Deep Copy
- Dynamic Memory Allocation (New, Delete)
Data Structures
A good algorithm usually comes together with a set of good data structures that allow the algorithm to manipulate the data efficiently. In this course, we MY TECH FOCUS consider the common data structures that are used in various computational problems. You will learn how these data structures are implemented in different programming languages and will practice implementing them in our programming assignments. This will help you to understand what is going on inside a particular built-in implementation of a data structure and what to expect from it. You will also learn typical use cases for these data structures.
- What is Data Structure?
- Why we need Data Structure?
- Stack
- Queue
- Linear Queue
- De-Queue
- Circular Queue
- Linked List
- Singly LinkedList
- Doubly LinkedList
- Circular LinkedList
- Searching
- Sorting
- Binary Tree